Understanding React Hooks: A Comprehensive Guide for Developers
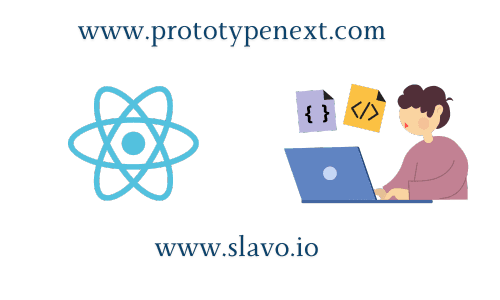
React has transformed how we build user interfaces by introducing a more declarative approach to UI development. One of the most significant updates to React is the introduction of hooks in version 16.8. Hooks provides a new way to use state and other React features without writing a class. In this blog post, we'll explore what hooks are, why we need them, how to create them, and delve into the most critical hooks in React. We'll also cover best practices to help you make the most out of hooks in your projects.
- React Key Concepts
- Mentorship & Consulting - Contact us for more info Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
What Are Hooks?
In React, hooks are special functions that allow you to "hook into" React state and lifecycle features from function components. They provide a powerful way to manage state, side effects, context, and other functionalities more concisely and reusable than was possible with class components. Let's delve into what hooks are, their significance, and the different types of hooks provided by React.
The Evolution of Hooks
Before hooks were introduced in React 16.8, developers used class components to manage state and lifecycle methods. Class components often led to complex and less readable code, significantly as applications grew in size and complexity. Sharing stateful logic between components took a lot of work, typically requiring higher-order components (HOCs) or render props, which could make the code harder to follow.
Hooks were introduced to address these pain points by enabling state and other React features in functional components. This shift has led to cleaner, more maintainable, and more reusable code.
Key Benefits of Hooks
-
Simplicity and Readability: Hooks allow you to manage state and side effects directly within functional components, reducing the need for boilerplate code and making components more accessible to read and understand.
-
Reusability: Custom hooks enable you to extract and reuse stateful logic across multiple components, promoting better code organization and reducing duplication.
-
Functional Programming: Hooks embraces functional programming principles, allowing you to write components as pure functions with minimal side effects.
-
Gradual Adoption: Hooks are backward-compatible and can be gradually adopted in existing codebases. You can start using hooks in new components without rewriting existing class components.
Rules of Hooks
To ensure consistent behavior and avoid pitfalls, React enforces two main rules when using hooks:
-
Only Call Hooks at the Top Level: Do not call hooks inside loops, conditions, or nested functions. Always use hooks at the top level of your React function to ensure they are called in the same order each time a component renders.
-
Only Call Hooks from React Functions: You can call hooks from React function components or custom hooks, but not from regular JavaScript functions.
Built-In Hooks
React provides several built-in hooks that cover everyday use cases for managing state, side effects, and context:
useState
The
Loading...
hook lets you add state to functional components. You pass the initial state toLoading...
, which returns an array with the current state and a function to update it.Loading...
useEffect
The
Loading...
hook allows you to perform side effects in function components, such as fetching data, directly interacting with the DOM, or setting up subscriptions. It runs after the render and can optionally clean up before the component unmounts.Loading...
useContext
The
Loading...
hook lets you subscribe to React context without introducing nesting, allowing you to access context values directly within function components.Loading...
useReducer
The
Loading...
hook is an alternative toLoading...
for managing more complex state logic. It is often used when the next state depends on the previous state or when you have multiple sub-values.Loading...
useCallback
The
Loading...
hook returns a memoized version of the callback function that only changes if one of the dependencies has changed. It is helpful for optimizing performance by preventing unnecessary re-renders.Loading...
useMemo
The
Loading...
hook memoizes a value, recomputing it only when one of the dependencies has changed. This can help optimize performance by avoiding expensive calculations on every render.Loading...
useRef
The
Loading...
hook allows you to persist values across renders without causing a re-render. It can also be used to access and manipulate DOM elements directly.Loading...
Custom Hooks
Custom hooks allow you to encapsulate and reuse stateful logic across multiple components. A custom hook is simply a JavaScript function that can call other hooks.
Loading...
By following these guidelines and understanding the core concepts of hooks, you can harness their full potential to write more efficient, maintainable, and scalable React applications.
Why Do We Need Hooks?
React hooks were introduced in version 16.8 to address several long-standing issues and limitations with class components. They provide a new way to manage state and other React features in functional components, leading to simpler, more maintainable, and reusable code. Here, we will explore why hooks are essential and how they improve the React development experience.
Simplifying Component Logic
One of the main reasons we need hooks is to simplify component logic. Before hooks, managing state and lifecycle methods in class components often led to complex and less readable code. Hooks allow you to organize related logic within a component more intuitively.
Class Component Example
Consider a class component that fetches data and manages a loading state:
Loading...
This code is verbose and mixes different concerns (fetching data and rendering) within the same class. Now, consider the same logic using hooks:
Functional Component with Hooks
Loading...
The functional component is more concise and separates concerns more cleanly, making the code easier to read and maintain.
Enhancing reusability
Sharing stateful logic between components in class components was challenging and often required higher-order components (HOCs) or render props. These patterns could lead to deeply nested components and less readable code. Hooks enable you to extract and reuse stateful logic without these drawbacks.
Custom Hooks for Reusability
Custom hooks allow you to encapsulate logic and reuse it across different components. For example, you can create a custom hook to handle fetching data:
Loading...
You can then use this custom hook in multiple components, promoting code reuse and reducing duplication:
Loading...
Improving performance
Hooks like
Loading...
andLoading...
help optimize performance by preventing unnecessary re-renders and recalculations. Memoizing functions and values in class components often require additional libraries or verbose code. Hooks make it easier to optimize performance directly within functional components.useCallback and useMemo
Loading...
In this example,
Loading...
memoizes theLoading...
function, andLoading...
memoizes the result of the expensive calculation. This prevents unnecessary recalculations and re-renders, improving performance.Embracing Functional Programming
Hooks embrace functional programming principles, allowing you to write components as pure functions with minimal side effects. This approach leads to more predictable and testable code. Functional components with hooks are more accessible to reason about, as they focus on inputs (props and state) and outputs (rendered UI) without managing internal state and lifecycle methods in a complex class structure.
Gradual Adoption
Hooks are backward-compatible and can be gradually adopted in existing codebases. You can start using hooks in new components or refactor existing components to use hooks without having to rewrite your entire codebase. This makes hooks a practical and non-disruptive addition to any React project.
Enhanced Developer Experience
Hooks improves the developer experience by making React code more intuitive and less verbose. The consistent patterns and more straightforward API reduce the learning curve for new developers and make code reviews and collaboration more straightforward.
React hooks have revolutionized how we write React applications by addressing the limitations of class components. They simplify component logic, enhance reusability, improve performance, embrace functional programming principles, and can be adopted gradually. By understanding the benefits and capabilities of hooks, you can write more efficient, maintainable, and scalable React applications, ultimately enhancing your development experience and productivity.
How to Create Hooks
React hooks provide a powerful and intuitive way to manage state and side effects in functional components. This section will cover using built-in hooks and creating custom hooks to encapsulate and reuse logic across your React components.
Using Built-In Hooks
React provides several built-in hooks that cover the most common use cases for managing state, side effects, and context. Below, we will explore some of the most commonly used built-in hooks.
useState
The
Loading...
hook adds a state to a functional component. It takes the initial state as an argument and returns an array with the current state and a function to update it.Loading...
In this example,
Loading...
initializes the state with a value ofLoading...
. TheLoading...
function updates the state when the button is clicked.useEffect
The
Loading...
hook allows you to perform side effects in your functional components. It takes a function as an argument, which React will call after the component renders. You can use this hook to fetch data, set up subscriptions, and more.Loading...
In this example,
Loading...
fetches data from the given URL whenever theLoading...
prop changes. The empty dependency arrayLoading...
means the effect runs only once when the component mounts.useContext
The
Loading...
hook lets you subscribe to React context without introducing nesting, allowing you to access context values directly within functional components.Loading...
In this example,
Loading...
retrieves the current value ofLoading...
, which is then used to set the button's background color.useReducer
The
Loading...
hook is an alternative toLoading...
for managing complex state logic. It is often used when the next state depends on the previous state or when you have multiple sub-values.Loading...
In this example,
Loading...
initializes the state and returns the current state. It also includes aLoading...
function to send actions to the reducer.Creating Custom Hooks
Custom hooks allow you to encapsulate and reuse stateful logic across multiple components. A custom hook is simply a JavaScript function that can call other hooks. By convention, custom hooks start with the word "use."
Example of a Custom Hook
Let's create a custom hook that fetches data from an API.
Loading...
In this custom hook,
Loading...
, we useLoading...
to manage the data and loading state andLoading...
to fetch the data when theLoading...
changes. The custom hook returns the data and loading state, making it easy to reuse this logic in different components.Using the Custom Hook
Loading...
Using the
Loading...
custom hook, we simplify theLoading...
component and reusable the data-fetching logic.Best Practices for Creating Hooks
-
Prefix with "use": Always start custom hooks with "use" to indicate that they follow the rules of hooks and can call other hooks.
-
Keep Hooks at the Top Level: Do not call hooks inside loops, conditions, or nested functions. Always use hooks at the top level of your React function to ensure consistent behavior.
-
Return a Consistent API: Custom hooks should return a consistent API that makes them easy to use and understand. Typically, this involves returning state variables and any functions needed to update the state.
-
Avoid Side Effects: Try to keep custom hooks pure and avoid side effects within the hook itself. If you need to perform side effects, use
Loading...
inside the hook. -
Use Descriptive Names: Name your custom hooks descriptively, clearly indicating their purpose and functionality.
Hooks have transformed how we write React components, making our code more concise, readable, and reusable. By mastering built-in hooks and creating custom hooks, you can leverage the full power of React to build efficient and maintainable applications. Whether you're managing state, side effects, or context, hooks provide a consistent and intuitive API that simplifies React development.
Best Practices for Using Hooks
React hooks provide a powerful and flexible way to manage state and side effects in functional components. However, to leverage their capabilities and avoid common pitfalls, it's essential to follow best practices. Here are some detailed best practices for using hooks in your React applications.
1. Follow the Rules of Hooks
Only Call Hooks at the Top Level
Hooks should always be at the top of your React function components, not inside loops, conditions, or nested functions. This ensures that hooks are called in the same order every time a component renders, which is crucial for React to manage state and side effects correctly.
Loading...
Only Call Hooks from React Functions
Hooks should only be called from React function components or custom hooks. Do not call hooks from regular JavaScript functions.
Loading...
2. Use Custom Hooks for Reusable Logic
Custom hooks allow you to encapsulate and reuse stateful logic across multiple components. If you repeat the same logic in different elements, consider extracting it into a custom hook.
Loading...
3. Keep Hooks Simple and Focused
Custom hooks should be simple and focused on a single responsibility. This makes them easier to understand, test, and reuse. Consider breaking it down into smaller hooks if a hook does too much.
Loading...
4. Optimize performance with useMemo and useCallback
Use
Loading...
andLoading...
to optimize performance by memoizing expensive calculations and functions. This prevents unnecessary re-renders and improves the efficiency of your components.Loading...
5. Clean Up Side Effects
When using
Loading...
to perform side effects, ensure you clean up subscriptions, event listeners, or any other resources to prevent memory leaks and unexpected behavior.Loading...
6. Handle Dependencies Properly
When using
Loading...
,Loading...
, orLoading...
, list all dependencies that affect the hook. This ensures that the hook runs correctly and avoids stale closures.Loading...
7. Avoid Overusing useEffect
While
Loading...
is powerful, avoid overusing it for logic that can be handled elsewhere. ComplexLoading...
logic sometimes indicates that the component's responsibilities are not well-separated, and the code may benefit from refactoring.8. Debugging with useDebugValue
For custom hooks, use
Loading...
to display a label in React DevTools. This makes it easier to debug and understand the hook's state.Loading...
9. Avoid Premature Optimization
While performance optimization is essential, avoid premature optimization. First, focus on writing clear and correct code. Use
Loading...
andLoading...
when identifying performance bottlenecks, but don't add them everywhere unnecessarily.10. Use TypeScript for Type Safety
If you're using TypeScript, leverage its type safety features to create more robust hooks. Type your custom hooks to ensure they return the expected values and accept the correct arguments.
Loading...
By following these best practices, you can harness the full potential of hooks in React, creating more efficient, maintainable, and scalable applications. Hooks simplify component logic, enhance reusability, and improve the developer experience, making them an essential tool in modern React development.
** Book Recommendation:
- React and React Native: A complete hands-on guide to modern web and mobile development with React.js, 3rd Edition
- React Key Concepts
- Pragmatic Programmer The: Your journey to mastery, 20th Anniversary Edition
Mentorship & Consulting - Contact us for more info
Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
For Consulting and Mentorship, feel free to contact slavo.io