Understanding useEffect in React: A Comprehensive Guide from Beginner to Advanced
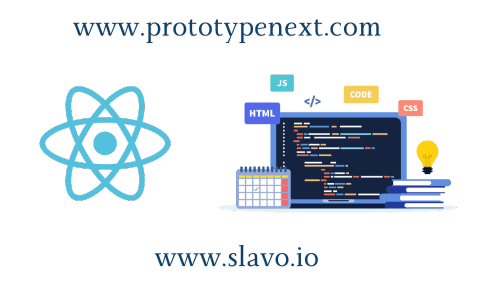
Understanding useEffect in React: A Comprehensive Guide from Beginner to Advanced
React's
Loading...
hook is a powerful tool that allows developers to perform side effects in functional components. This blog post will delve deep into theLoading...
hook, covering everything from the basics to advanced usage, helping you understand how to leverage it effectively in your React applications.Table of Contents
- Introduction to useEffect
- Basic Usage
- Dependency Array
- Cleaning Up Effects
- Conditional Effects
- Optimization Tips
- Advanced Patterns
- Common Pitfalls and Best Practices
1. Introduction to useEffect
What is useEffect?
Loading...
is a hook provided by React that allows you to perform side effects in your function components. Introduced in React 16.8,Loading...
has become an essential tool for managing side effects, replacing the need for class component lifecycle methods such asLoading...
,Loading...
, andLoading...
.What are Side Effects?
In the context of React, side effects are operations that occur outside the scope of rendering your component. These operations can include:
- Data Fetching: Making HTTP requests to fetch data from an API.
- Subscriptions: Setting up subscriptions to external data sources or events.
- Timers: Using
Loading...
orLoading...
to handle time-based operations. - Manual DOM Manipulations: Interacting with the DOM directly, such as adding event listeners or manipulating DOM nodes.
Why useEffect?
The primary reasons for using
Loading...
are:- Separation of Concerns:
Loading...
allows you to separate your side effect logic from your rendering logic, making your components more readable and maintainable. - Unified API: With
Loading...
, you can handle all side effect scenarios (mount, update, and unmount) within a single function, simplifying the mental model required to manage component lifecycle events. - Functional Components: Before hooks, side effects were managed using lifecycle methods in class components. Hooks, including
Loading...
, empower you to use functional components exclusively, which are often easier to read and test.
Basic Syntax
The
Loading...
hook takes two arguments:- Effect Function: A function that contains the side effect logic. This function is executed after the component renders.
- Dependency Array (optional): An array of dependencies that control when the effect runs. If not provided, the effect will run after every render.
Here's the basic syntax of
Loading...
:Loading...
Breakdown of the Example
- Effect Function: The first argument to
Loading...
is a function that runs the side effect. In the example above,Loading...
runs after the component renders or updates. - Cleanup Function: The optional return function inside the effect is a cleanup function that runs before the component unmounts or before the effect is re-executed on subsequent renders. In the example,
Loading...
runs during cleanup. - Dependency Array: The second argument is a dependency array that determines when the effect runs. An empty array (
Loading...
) means the effect runs only once, after the initial render.
Key Points to Remember
- Single Effect for All Lifecycle Phases:
Loading...
can handle mounting, updating, and unmounting, reducing the need for separate lifecycle methods. - Dependency Management: Managing the dependency array is crucial for performance and avoiding unnecessary re-renders.
- Cleanup Logic: Always provide cleanup logic for effects that create resources (like subscriptions or timers) to prevent memory leaks.
The
Loading...
hook is a versatile and powerful tool for managing side effects in React functional components. By understanding its basic syntax and usage, you can leverageLoading...
to handle side effects efficiently in your applications. The upcoming sections will dive deeper into more advanced usage patterns, optimization techniques, and common pitfalls to watch out for, helping you masterLoading...
and build robust React components.2. Basic Usage of useEffect
The
Loading...
hook in React is designed to handle side effects in functional components. Let's explore its primary usage with practical examples and detailed explanations to build a solid understanding.Setting Up a Basic useEffect
The simplest form of
Loading...
involves using it without any dependencies. In this case, the effect runs after every render of the component.Loading...
In this example:
Loading...
logs a message every time the component renders or updates.- The effect runs after every render because no dependency array is provided.
Running an Effect Only Once (On Mount)
When the component is mounted, you provide an empty dependency array (
Loading...
) to run an effect only once. This ensures the impact runs only after the initial render.Loading...
In this example:
- The effect logs a message only once, after the initial render.
- The empty dependency array (
Loading...
) ensures the effect doesn't run on subsequent renders.
Running an Effect When Dependencies Change
You can specify dependencies in the array to control when an effect runs. The effect runs only when any of the dependencies change.
Loading...
In this example:
- The effect logs a message every time
Loading...
changes. - The dependency array
Loading...
ensures the effect runs only whenLoading...
updates.
Cleaning Up Effects
When an effect creates resources that need to be cleaned up, such as subscriptions or timers, you can return a cleanup function from the impact. Due to dependency changes, this function runs before the component unmounts or the effect re-executes.
Loading...
In this example:
- The effect sets up a timer that logs a message every second.
- The cleanup function clears the timer when the component unmounts or before the effect re-executes.
- This prevents memory leaks by ensuring the timer is appropriately cleaned up.
Combining Multiple Effects
You can use multiple
Loading...
hooks in a single component to handle different side effects separately. This helps in keeping your code organized and easier to manage.Loading...
In this example:
- One
Loading...
runs whenLoading...
changes, and another runs whenLoading...
changes. - This separation of concerns makes the component logic more transparent and maintainable.
Understanding the primary usage of
Loading...
is crucial for managing side effects in React functional components. By mastering the different ways to useLoading...
—running effects after every render, only once on mount, or when dependencies change—you can efficiently handle various side effects in your applications. Remember to clean up resources to avoid memory leaks and feel free to use multipleLoading...
hooks to keep your code organized. As you become more comfortable withLoading...
, you can explore advanced patterns and optimizations to further enhance your React components.3. Dependency Array in useEffect
The dependency array is a crucial aspect of the
Loading...
hook in React. It allows you to control when your side effect runs, optimizing performance and avoiding unnecessary operations. This section will explore how the dependency array works, its significance, and how to use it effectively.What is the Dependency Array?
The dependency array is the second argument passed to the
Loading...
hook. It is an array of values on which the effect depends. React uses this array to determine when to re-run the effect. By specifying dependencies, you can control how frequently the effect executes.Different Scenarios with the Dependency Array
- No Dependency Array
- Empty Dependency Array
- Dependencies Specified
1. No Dependency Array
When you omit the dependency array, the effect runs after every component is rendered. This can be useful for logging or debugging purposes but is generally not recommended for side effects that can be expensive or should only run under specific conditions.
Loading...
In this example:
- The effect runs after every render, regardless of any state or prop changes.
- This can lead to performance issues if the effect is computationally intensive.
2. Empty Dependency Array
An empty dependency array (
Loading...
) means the effect runs only once, after the initial render. This is equivalent to theLoading...
lifecycle method in class components.Loading...
In this example:
- The effect runs only once after the component is mounted.
- This is useful for initializing data, setting up subscriptions, or performing one-time side effects.
3. Dependencies Specified
Specifying dependencies in the array allows the effect to run only when those dependencies change. This is essential for optimizing performance and ensuring the effect runs only when necessary.
Loading...
In this example:
- The effect runs only when the
Loading...
state changes. - This helps in avoiding unnecessary effect executions and improves performance.
Multiple Dependencies
You can specify multiple dependencies in the array. The effect runs when any of the dependencies change.
Loading...
In this example:
- The effect runs when either
Loading...
orLoading...
changes. - This ensures that the effect is synchronized with both pieces of state.
Handling Objects and Arrays as Dependencies
When using objects or arrays as dependencies, be cautious. React performs a shallow comparison of dependencies. If an object or array is recreated on every render, the effect will run on every render, even if the values inside the object or array haven't changed.
Loading...
In this example:
- The effect runs on every render because the
Loading...
object is recreated each time. - To avoid this, ensure the dependency doesn't change unless necessary.
Loading...
In this optimized example:
- The effect runs only when the
Loading...
state changes.
Best Practices
- Minimize Dependencies: Include only the necessary dependencies to avoid unnecessary re-renders.
- Memoization: Use
Loading...
orLoading...
to memoize functions or values to prevent unnecessary changes. - Stable References: Ensure the dependencies are stable and don't change unless required.
The dependency array in
Loading...
is a powerful tool for controlling when your side effects run. By understanding and leveraging them effectively, you can optimize your React components for better performance and cleaner code. Always remember to carefully manage your dependencies to ensure that your effects run at the appropriate times, avoiding unnecessary executions and potential performance issues.4. Cleaning Up Effects in useEffect
Cleaning up effects is crucial to using the
Loading...
hook in React. When your effect creates resources that need to be cleaned up, such as subscriptions, timers, or event listeners, it's essential to clean up these resources to prevent memory leaks and unwanted behaviors.Why Clean Up?
Cleaning up effects ensures that you:
- Prevent Memory Leaks: Avoid retaining resources no longer needed.
- Maintain Performance: Free up resources to keep your application running smoothly.
- Ensure Correctness: Avoid side effects from previous renders affecting current renders.
How to Clean Up Effects
You can clean up effects by returning a cleanup function from the effect function. This cleanup function occurs when the component unmounts or before the effect re-executes due to dependency changes.
Basic Cleanup Example
Let's start with a simple example using a timer.
Loading...
In this example:
- The effect sets up a timer that logs a message every second.
- The cleanup function,
Loading...
, stops the timer when the component unmounts or before the effect runs again.
Cleaning Up Subscriptions
If your effect involves setting up subscriptions, such as a WebSocket or event listener, you should clean up the subscription to prevent memory leaks.
Loading...
In this example:
- The effect sets up an event listener for the
Loading...
event on the window. - The cleanup function,
Loading...
, removes the event listener when the component unmounts or before the effect re-runs.
Cleaning Up Fetch Requests
When working with fetch requests, you should clean up to prevent state updates on unmounted components. You can do this using the AbortController.
Loading...
In this example:
- The effect fetches data from an API.
- The cleanup function,
Loading...
, cancels the fetch request if the component unmounts or before the effect runs again.
Dependencies and Cleanup
The cleanup function runs before the effect re-executes due to dependency changes. This ensures that any ongoing operations from the previous impact are cleaned up before starting new ones.
Loading...
In this example:
- The effect sets a timer based on the
Loading...
state. - The cleanup function clears the previous timer before setting up a new one whenever
Loading...
changes.
Best Practices for Cleaning Up Effects
- Always Clean Up: Ensure you always provide a cleanup function when your effect sets up resources that need to be cleaned up.
- Use Proper Dependencies: Include all necessary dependencies in the array to avoid missing cleanup for changing values.
- Handle Async Operations: To handle async operations properly, Use mechanisms like AbortController for fetch requests.
Cleaning up effects is essential to using the
Loading...
hook in React. By adequately managing cleanup, you can prevent memory leaks, maintain performance, and ensure the Correctness of your application. Always return a cleanup function for effects that set up resources, and carefully manage dependencies to ensure your impact runs and cleans up as expected. With these practices, you can write robust and efficient React components that handle side effects gracefully.5. Conditional Effects
In React,
Loading...
allows you to perform side effects in functional components, and often you need to run these effects conditionally. Understanding how to implement conditional effects properly ensures that your components behave as expected and are optimized for performance.What Are Conditional Effects?
Conditional effects refer to running an effect only under certain conditions. This can involve:
- Running an effect based on the presence of certain data.
- Skipping an effect when a particular condition is not met.
- Running different effects based on varying conditions.
Basic Conditional Effect
To conditionally run an effect, you can place conditional logic inside the effect function or control the dependencies in the dependency array. Here’s an example of using conditional logic inside the effect:
Loading...
In this example:
- The effect runs every time
Loading...
changes. - Inside the effect, there is a condition to log a message only when
Loading...
is even.
Using Early Return for Conditional Effects
Another way to handle conditional effects is by using early return statements. This can make your effect functions more readable, especially with complex conditions.
Loading...
In this example:
- The effect runs only if
Loading...
is not null. - An early return statement skips the effect when
Loading...
is null.
Conditional Data Fetching
A common use case for conditional effects is data fetching, where data is only fetched when certain conditions are met.
Loading...
In this example:
- Data is fetched only when
Loading...
is provided. - The effect and data fetch are skipped if
Loading...
is null or undefined.
Combining Multiple Conditions
Sometimes, you may need to combine multiple conditions to determine when an effect should run. This can be achieved by incorporating all necessary checks within the effect function.
Loading...
In this example:
- The effect runs only if both
Loading...
andLoading...
are provided. - Multiple conditions are checked before running the effect.
Best Practices for Conditional Effects
- Minimize Dependencies: Only include necessary dependencies to avoid unnecessary re-renders.
- Use Early Returns: Early return statements can simplify your effect logic and make it more readable.
- Combine Conditions: When necessary, combine multiple conditions to control when the effect should run.
- Avoid Complex Logic in Effects: Move complex logic outside the effect function to keep it clean and maintainable.
- Test Thoroughly: Ensure that all conditions are correctly handled and that your effects run as expected in all scenarios.
Conditional effects in React using
Loading...
are essential for creating efficient and responsive components. By carefully managing when your effects run, you can optimize performance and ensure your components behave correctly. Utilize early returns, combine conditions, and follow best practices to master conditional effects in your React applications.6. Optimization Tips for useEffect
Optimizing the use of
Loading...
in your React components can significantly improve performance and maintainability. Here are some tips and techniques to help you optimize your effects effectively.1. Minimize Dependencies
One of the most critical optimization strategies is to minimize the dependencies you include in the dependency array. Including only necessary dependencies ensures that the effect runs only when needed, reducing unnecessary re-renders and performance overhead.
Loading...
In this example:
- The effect runs only when the
Loading...
state changes, ignoring changes to theLoading...
state.
2. Memoize Callbacks and Values
Use
Loading...
andLoading...
to memoize the functions and values used in your effects. Memoization ensures that functions and values only change when necessary, preventing unnecessary effect executions.Loading...
In this example:
- The
Loading...
value is memoized and only recalculated whenLoading...
changes. - The
Loading...
function is memoized and does not change unless necessary, reducing unnecessary effect executions.
3. Debounce Effects
For effects that respond to rapid changes, such as user input, debounce the impact to reduce the number of executions. This is particularly useful for search inputs or API calls.
Loading...
In this example:
- The input value is debounced with a delay of 300 milliseconds, reducing the number of effect executions and API calls.
4. Split Effects
Split complex effects into multiple, more straightforward effects. This makes your code easier to understand, test, and maintain. Each effect should handle a single responsibility.
Loading...
In this example:
- One effect handles logging changes to
Loading...
. - Another effect handles data fetching, ensuring each has a single responsibility.
5. Avoid Inline Functions and Objects
Avoid passing inline functions and objects as dependencies. Inline values are recreated on every render, unnecessarily causing the effect to run.
Loading...
In this example:
- The
Loading...
function is memoized usingLoading...
, preventing it from being recreated on every render.
6. Use React DevTools Profiler
Use the React DevTools Profiler to analyze the performance of your components and effects. The Profiler helps you identify which components re-render unnecessarily and optimize accordingly.
- Install React DevTools: Install the React DevTools extension for your browser.
- Profile Your Application: Use the Profiler tab to record and analyze performance.
- Identify Bottlenecks: Look for components with frequent re-renders and optimize their effects.
Optimizing the use of
Loading...
in your React applications can significantly improve performance and maintainability. By minimizing dependencies, memoizing callbacks and values, debouncing effects, splitting complex effects, avoiding inline functions, and using the React DevTools Profiler, you can ensure your effects run efficiently and only when necessary. Implement these optimization tips to create more responsive and performant React applications.7. Advanced Patterns with useEffect
Once you are comfortable with the basics of
Loading...
, you can explore more advanced patterns to handle complex scenarios and optimize your React components further. This section covers advanced patterns such as synchronizing effects with props, custom hooks for reusable logic, handling async operations, and more.1. Synchronizing Effects with Props
Sometimes, you need to synchronize effects with changes in props. This can be useful when fetching data based on prop changes or updating the state when a prop changes.
Loading...
In this example:
- The effect fetches user data whenever the
Loading...
prop changes. - The effect does nothing if
Loading...
is not provided.
2. Custom Hooks for Reusable Logic
Custom hooks allow you to extract reusable logic from your components, making your code more modular and easier to maintain. You can create custom hooks that use
Loading...
internally.Loading...
You can use this custom hook in your components:
Loading...
In this example:
- The custom hook
Loading...
encapsulates the data fetching logic. - The component
Loading...
uses the custom hook to fetch and display data.
3. Handling Async Operations
When handling async operations in
Loading...
it's important to handle potential race conditions and cancellations. Using anLoading...
or a similar mechanism can help manage async operations effectively.Loading...
In this example:
- The
Loading...
cancels the fetch request if the component unmounts or the effect re-runs. - This prevents potential race conditions and ensures the component state is only updated when appropriate.
4. Combining Multiple Effects
You may need to combine multiple effects to handle different side effects in more complex components. Keeping each effect focused on a single responsibility can make your code more maintainable and accessible to debug.
Loading...
In this example:
- One effect handles logging changes to the
Loading...
state. - Another effect handles fetching data when the component mounts.
5. Using Effect Cleanup Functions
Cleanup functions ensure that resources allocated by an effect are released appropriately. This is especially important for subscriptions, timers, or any other resource that needs explicit cleanup.
Loading...
In this example:
- A subscription is set up when the component mounts.
- The cleanup function ensures that the subscription is unsubscribed correctly when the component unmounts or
Loading...
changes.
Advanced patterns with
Loading...
allow you to handle more complex scenarios in your React applications. You can create robust and optimized components by synchronizing effects with props, creating custom hooks, managing async operations effectively, combining multiple effects, and utilizing cleanup functions. These patterns help you write cleaner, more maintainable code and ensure your components perform well under various conditions. As you gain experience, experimenting with these advanced patterns will further enhance your skills in managing side effects in React.8. Common Pitfalls and Best Practices
Using
Loading...
effectively in React can be challenging, especially when dealing with complex logic and dependencies. Understanding common pitfalls and following best practices can help you avoid bugs, improve performance, and write more maintainable code.Common Pitfalls
- Unnecessary Re-renders
- Missing Dependencies
- Incorrect Cleanup
- Running Effects Conditionally
- Race Conditions in Async Operations
1. Unnecessary Re-renders
Unnecessary re-renders occur when your effect runs more often than needed. This usually happens due to incorrect dependencies or inline functions and objects.
Example of Unnecessary Re-renders:
Loading...
Solution:
Provide a dependency array to control when the effect should run.
Loading...
2. Missing Dependencies
A common mistake is to omit dependencies that should be included, leading to stale closures and unexpected behavior.
Example of Missing Dependencies:
Loading...
Solution:
Include all relevant dependencies in the dependency array.
Loading...
3. Incorrect Cleanup
Failing to clean up resources properly can lead to memory leaks and unexpected behavior, especially with subscriptions, timers, or event listeners.
Example of Incorrect Cleanup:
Loading...
Solution:
Always provide a cleanup function to release resources.
Loading...
4. Running Effects Conditionally
Running effects conditionally within the effect function can lead to inconsistencies and hard-to-debug issues.
Example of Running Effects Conditionally:
Loading...
Solution:
Include the condition in the dependency array and handle the logic inside the effect.
Loading...
5. Race Conditions in Async Operations
Race conditions can occur when dealing with async operations if previous operations are not appropriately canceled.
Example of Race Conditions:
Loading...
Solution:
Use an
Loading...
to handle cancellations.Loading...
Best Practices
- Always Specify Dependencies
- Use Custom Hooks for Reusable Logic
- Keep Effects Simple and Focused
- Use Memoization to Prevent Unnecessary Effects
- Handle Cleanup Correctly
1. Always Specify Dependencies
Specify all dependencies in the dependency array to avoid stale closures and ensure your effects run correctly.
Loading...
2. Use Custom Hooks for Reusable Logic
Encapsulate reusable logic in custom hooks to keep your components clean and focused.
Loading...
3. Keep Effects Simple and Focused
Keep your effects simple and focused on a single responsibility. If an effect becomes too complex, consider splitting it into multiple effects.
Loading...
4. Use Memoization to Prevent Unnecessary Effects
Use
Loading...
andLoading...
to memoize values and functions, preventing unnecessary re-renders and effect executions.Loading...
5. Handle Cleanup Correctly
Always return a cleanup function from your effect to release resources appropriately.
Loading...
By understanding and avoiding common pitfalls and following best practices, you can use the
Loading...
hook more effectively in your React applications. Proper dependency management, cleanup, memoization, and keeping your effects simple and focused will help you write robust and performant components. Implement these practices to ensure your components behave as expected and are easier to maintain and debug.React's
Loading...
hook is essential for performing side effects in functional components. By understanding its syntax, dependency management, cleanup mechanisms, and advanced patterns, you can harness its full potential to build robust, efficient React applications.Remember, the key to mastering
Loading...
lies in practice and experience. Experiment with different scenarios, debug your effects, and continually refine your approach to achieve optimal results.Feel free to ask any questions or suggest topics for future posts. Happy coding!
** Book Recommendation:
Mentorship & Consulting - Contact us for more info
Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
For Consulting and Mentorship, feel free to contact slavo.io