Understanding Object Types in TypeScript: A Comprehensive Guide
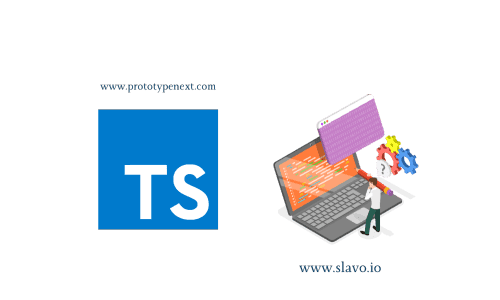
TypeScript, a statically typed superset of JavaScript, has gained immense popularity among developers for its ability to catch errors early and enhance code maintainability. One of the core aspects of TypeScript is its robust type system, which includes various ways to define object types. This article will dive deep into the different object types in TypeScript, including nested types, providing a thorough understanding to help you write more reliable and readable code.
Basic Object Types in TypeScript: Interfaces and Types
TypeScript provides two main ways to define object types: using
Loading...
and usingLoading...
aliases. Both approaches have strengths and are often used interchangeably. This section will explore how to define basic object types using bothLoading...
andLoading...
aliases, their differences, and their use cases.** Book Recommendation:
- React and React Native: A complete hands-on guide to modern web and mobile development with React.js, 3rd Edition
- React Key Concepts
- Pragmatic Programmer The: Your journey to mastery, 20th Anniversary Edition
Mentorship & Consulting - Contact us for more info
Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
Defining Object Types with Interfaces
An
Loading...
in TypeScript is a powerful way to define the shape of an object. It provides a clear and concise way to specify what properties an object should have, including their types.Loading...
In this example, we define an
Loading...
namedLoading...
with two properties:Loading...
of typeLoading...
andLoading...
of typeLoading...
. The objectLoading...
must adhere to this shape, ensuring type safety.Defining Object Types with Type Aliases
A
Loading...
alias can also be used to define the shape of an object. WhileLoading...
aliases can do more than define object shapes (they can define union types, intersection types, etc.), they are often used similarly to interfaces for object definitions.Loading...
Here, we use a
Loading...
alias to define the sameLoading...
object type as before. The result is identical to using anLoading...
.Differences Between Interfaces and Type Aliases
While
Loading...
andLoading...
aliases are similar, they have some differences:- Extending and Implementing:
Loading...
can be extended and implemented by other interfaces and classes.Loading...
aliases can be intersected using theLoading...
operator but cannot be implemented by classes.
Loading...
In this example, the
Loading...
interface extends theLoading...
interface, inheriting its properties.With
Loading...
aliases, we achieve a similar result using intersection types:Loading...
- Declaration Merging:
Loading...
support declaration merging, meaning you can define multipleLoading...
declarations with the same name, and TypeScript will merge them.Loading...
aliases do not support declaration merging.
Loading...
In this example, the
Loading...
interface declarations are merged into one, containing both theLoading...
andLoading...
properties.Using Interfaces and Type Aliases Together
Using both
Loading...
andLoading...
aliases in the same codebase is common. Here’s an example where we define aLoading...
type and extend it using an interface:Loading...
In this example, the
Loading...
type is defined using aLoading...
alias, and theLoading...
interface includes it as a property. This approach showcases how you can leverage bothLoading...
andLoading...
aliases to structure your types effectively.Defining object types is a fundamental part of working with TypeScript. Whether you use
Loading...
orLoading...
aliases, both offer powerful ways to describe the shape and structure of objects in your code. Understanding their similarities and differences will help you choose the right tool for each situation, leading to more robust and maintainable TypeScript applications.Nested Object Types in TypeScript
Nested object types are essential in TypeScript for modeling more complex data structures. They allow us to define objects within objects, providing a way to describe hierarchical data accurately. In this section, we'll explore how to create nested object types using both
Loading...
andLoading...
aliases and examples to illustrate their usage.Defining Nested Object Types with Interfaces
Using
Loading...
, we can define a nested object type by specifying an object property whose type is anotherLoading...
.Loading...
In this example:
- The
Loading...
interface describes the structure of an address. - The
Loading...
interface includes anLoading...
property of typeLoading...
, making it a nested type. - The
Loading...
object must conform to theLoading...
interface, including the nestedLoading...
property.
Defining Nested Object Types with Type Aliases
Type aliases can also be used to define nested object types similarly.
Loading...
Here, we achieve the same structure using
Loading...
aliases. TheLoading...
type alias defines the address structure, and theLoading...
type alias includes anLoading...
property of typeLoading...
.Optional Nested Properties
In real-world applications, some properties might be optional. TypeScript allows us to mark nested properties as optional using the
Loading...
operator.Loading...
In this example:
- The
Loading...
property in theLoading...
interface is optional. - The
Loading...
property in theLoading...
interface is also optional. - The
Loading...
object can include these properties, but they are not required.
Readonly Nested Properties
TypeScript allows us to define
Loading...
properties within nested object types to ensure they cannot be modified after initialization.Loading...
In this example, the
Loading...
property in theLoading...
interface isLoading...
, preventing any modifications after theLoading...
object is initialized.Recursive Nested Types
Recursive types help define tree-like structures where a type references itself. This can be achieved using both
Loading...
andLoading...
aliases.Using
Loading...
:Loading...
Using
Loading...
aliases:Loading...
In both examples, the
Loading...
type includes an optionalLoading...
property, an array ofLoading...
objects. This recursive structure allows us to define nested categories.Nested object types in TypeScript provide a powerful way to model complex data structures, ensuring type safety and clarity in your code. Whether you use
Loading...
orLoading...
aliases, understanding how to define and work with nested types is essential for building robust TypeScript applications. By leveraging optional properties,Loading...
properties, and recursive types, you can create flexible and maintainable data models that accurately represent your application's requirements.Optional Properties in TypeScript
Optional properties in TypeScript are a powerful feature that allows you to define object properties that may or may not be present. This is particularly useful for modeling real-world scenarios where specific data might be optional or dependent on other factors. In this section, we'll explore how to define and work with optional properties using both
Loading...
andLoading...
aliases, along with examples to illustrate their usage.Defining Optional Properties with Interfaces
To define an optional property in an
Loading...
, you use theLoading...
operator after the property name. This indicates that the property is not required.Loading...
In this example:
- The
Loading...
interface defines two properties:Loading...
andLoading...
. - The
Loading...
property is optional, meaning an object conforming to theLoading...
interface can either include or omit it. Loading...
includes bothLoading...
andLoading...
, whileLoading...
only includesLoading...
.
Defining Optional Properties with Type Aliases
Type aliases can also be used to define similar optional properties.
Loading...
The
Loading...
type alias defines the same structure with an optionalLoading...
property. The behavior is identical to using anLoading...
.Optional Nested Properties
You can also define optional properties within nested objects. This is useful for complex data structures where specific nested properties might be optional.
Using
Loading...
:Loading...
In this example:
- The
Loading...
interface has an optionalLoading...
property. - The
Loading...
interface has optionalLoading...
andLoading...
properties. Loading...
includes all properties exceptLoading...
.Loading...
includes all properties.Loading...
only includes theLoading...
property.
Using
Loading...
aliases:Loading...
Here, we achieve the same result using
Loading...
aliases. The optional properties work the same way as withLoading...
.Optional Properties in Function Parameters
Optional properties can also be used in function parameters for more flexible function signatures.
Loading...
In this example, the
Loading...
function accepts aLoading...
object and constructs a greeting message. If theLoading...
property is present, it includes the age in the message; otherwise, it consists of the name.Optional Properties with Default Values
When using optional properties, you should provide default values. This can be achieved using object destructuring and default values in function parameters.
Loading...
In this example:
- The
Loading...
function accepts aLoading...
object and assigns a default value ofLoading...
toLoading...
if it is not provided. Loading...
has an explicitly providedLoading...
, whileLoading...
uses the default value.
Optional properties in TypeScript provide a flexible way to define object structures that can adapt to varying data requirements. You can create more adaptable and resilient code by understanding how to use optional properties with
Loading...
,Loading...
aliases, and function parameters. Whether modeling simple objects or complex nested structures, optional properties help you manage optional data cleanly and effectively.Readonly Properties in TypeScript
Readonly properties in TypeScript are a powerful feature that ensures specific properties of an object cannot be modified once they are assigned. This immutability can be crucial for maintaining data integrity, especially in more extensive and complex applications. In this section, we’ll explore how to define and work with readonly properties using both
Loading...
andLoading...
aliases, along with examples to illustrate their usage.Defining Readonly Properties with Interfaces
To define a readonly property in an
Loading...
, you use theLoading...
modifier before the property name. This indicates that the property can be set when the object is first created but cannot be changed afterward.Loading...
In this example:
- The
Loading...
interface defines a readonlyLoading...
property. - The
Loading...
property is set when theLoading...
object is created. - Any attempt to change the
Loading...
property afterward will result in a compilation error.
Defining Readonly Properties with Type Aliases
Type aliases can also be used to define readonly properties similarly.
Loading...
Here, we achieve the same result using a
Loading...
alias. TheLoading...
modifier ensures theLoading...
property cannot be changed after the initial assignment.Readonly Nested Properties
Readonly properties can also be defined within nested objects, ensuring that nested data remains immutable.
Using
Loading...
:Loading...
Using
Loading...
aliases:Loading...
In these examples:
- The
Loading...
property in theLoading...
type is readonly. - Any attempt to change the
Loading...
property after the initial assignment will result in a compilation error.
Readonly Arrays
TypeScript also supports read-only arrays, which ensure the array's contents cannot be modified after creation. This is useful for collections that should remain constant.
Loading...
In this example:
- The
Loading...
array is declared aLoading...
array. - Any attempt to modify the array (e.g., using
Loading...
or direct assignment) will result in a compilation error.
Readonly Tuples
Readonly tuples work similarly to readonly arrays, providing immutability for fixed-length collections.
Loading...
In this example:
- The
Loading...
tuple is declared asLoading...
. - Any attempt to modify the tuple will result in a compilation error.
Immutability with
Loading...
TypeScript provides a built-in utility type,
Loading...
, which can make all properties of a type readonly. This is especially useful for making existing types immutable without modifying their original definitions.Loading...
In this example:
- The
Loading...
type makes all properties of theLoading...
type readonly. - Any attempt to modify the properties of the
Loading...
object will result in a compilation error.
Readonly properties in TypeScript provide a way to enforce immutability, ensuring that specific properties cannot be modified after they are initially set. This can help prevent bugs and maintain data integrity, especially in larger applications where data consistency is crucial. By understanding how to define and use readonly properties with
Loading...
,Loading...
aliases, readonly arrays, readonly tuples, and theLoading...
utility type, you can create more robust and reliable TypeScript code.Index Signatures in TypeScript
Index signatures in TypeScript allow you to define object types with dynamically named properties. They are instrumental when you need to know the exact names of the properties in advance and the shape and type of the values those properties will hold. This section will explore how to define and use index signatures, along with examples to illustrate their usage.
Defining Index Signatures with Interfaces
An index signature can be added to an
Loading...
to specify that an object can have properties with arbitrary names, but the values must follow a specified type.Loading...
In this example:
- The
Loading...
interface defines an index signatureLoading...
. - This means any property with a string key must have a string value.
- The
Loading...
object adheres to this interface, allowing for dynamically named properties.
Defining Index Signatures with Type Aliases
Type aliases can also be used to define index signatures similarly.
Loading...
Here, the
Loading...
type alias defines the same structure as the interface example, allowing for dynamically named string properties.Index Signatures with Number Keys
Index signatures can also use number keys. This is useful for objects where the property names are numbers.
Loading...
In this example:
- The
Loading...
interface defines an index signatureLoading...
. - This means any property with a number key must have a string value.
- The
Loading...
object adheres to this interface, allowing for numerically indexed properties.
Combining Index Signatures with Known Properties
Index signatures can be combined with known properties to allow for objects with a mix of known and dynamic properties.
Loading...
In this example:
- The
Loading...
interface defines a known propertyLoading...
and an index signatureLoading...
. - The
Loading...
object adheres to this interface, allowing for known and dynamically named properties.
Index Signatures with Complex Types
Index signatures can also specify more complex value types, including objects and arrays.
Loading...
In this example:
- The
Loading...
interface defines an index signatureLoading...
. - This means any property with a string key must have an object value with
Loading...
andLoading...
properties. - The
Loading...
object adheres to this interface, allowing for complex dynamic properties.
Using Index Signatures with TypeScript's Utility Types
TypeScript provides utility types that can work with index signatures. For instance, you can use
Loading...
to make all properties optional.Loading...
In this example:
- The
Loading...
type makes all properties ofLoading...
optional. - The
Loading...
object can have any subset of properties defined inLoading...
.
Index signatures in TypeScript provide a flexible way to define object types with dynamic property names. You can create objects that accommodate known and dynamic properties by understanding how to use index signatures with
Loading...
andLoading...
aliases. Whether dealing with simple string or number keys or more complex value types, index signatures help you manage dynamic data structures effectively and safely.Intersection Types in TypeScript
Intersection types in TypeScript provide a powerful way to combine multiple types into one. They allow you to create a new type with all the properties of the intersected types, enabling you to model more complex data structures and ensure type safety in your applications. This section will explore how to define and use intersection types and examples to illustrate their usage.
Defining Intersection Types
Intersection types are defined using the
Loading...
operator. They can combine multiple object types, primitive types, or other intersection types.Loading...
In this example:
- The
Loading...
interface defines a type withLoading...
andLoading...
properties. - The
Loading...
interface defines a type withLoading...
andLoading...
properties. - The
Loading...
type intersects withLoading...
andLoading...
, combining all properties from both interfaces. - The
Loading...
object must conform to theLoading...
type, ensuring it has all properties fromLoading...
andLoading...
.
Combining Type Aliases with Intersection Types
Intersection types can also be used with type aliases to combine different shapes.
Loading...
In this example:
- The
Loading...
type alias defines a type withLoading...
,Loading...
, andLoading...
properties. - The
Loading...
type alias defines a type withLoading...
andLoading...
properties. - The
Loading...
type intersects withLoading...
andLoading...
combining all properties from both type aliases. - The
Loading...
object must conform to theLoading...
type, ensuring it has all properties fromLoading...
andLoading...
.
Using Intersection Types with Classes
Intersection types can also be used with classes, combining the properties and methods of multiple courses into one kind.
Loading...
In this example:
- The
Loading...
class hasLoading...
andLoading...
properties. - The
Loading...
class hasLoading...
andLoading...
properties. - The
Loading...
type is an intersection ofLoading...
andLoading...
, combining all properties from both classes. - The
Loading...
object must conform to theLoading...
type, ensuring it has all properties fromLoading...
andLoading...
.
Handling Conflicts in Intersection Types
When combining types with intersection types, handling potential conflicts between properties with the same name but different types is important.
Loading...
In this example:
- The
Loading...
interface defines aLoading...
property of typeLoading...
. - The
Loading...
interface defines aLoading...
property of typeLoading...
. - The
Loading...
type intersectsLoading...
andLoading...
. - Since
Loading...
cannot be bothLoading...
andLoading...
simultaneously, TypeScript infers the type ofLoading...
asLoading...
, leading to a type conflict.
To resolve such conflicts, the types must be redesign to avoid overlapping property names or use type unions instead.
Using Intersection Types with Utility Types
TypeScript provides several utility types that can work with intersection types, allowing for more advanced type manipulations.
Loading...
In this example:
- The
Loading...
interface defines aLoading...
property of typeLoading...
. - The
Loading...
interface defines aLoading...
property of typeLoading...
. - The
Loading...
type is an intersection ofLoading...
andLoading...
. - The
Loading...
type makes all properties ofLoading...
readonly using theLoading...
utility type. - Any attempt to modify the properties of
Loading...
will result in a compilation error.
Intersection types in TypeScript provide a versatile way to combine multiple types into one, enabling you to model complex data structures and ensure comprehensive type safety. By understanding how to use intersection types with
Loading...
,Loading...
aliases, classes, and utility types, you can create more flexible and robust TypeScript applications. Whether handling simple type combinations or resolving property conflicts, intersection types help you manage your data structures effectively and accurately.Union Types in TypeScript
Union types in TypeScript allow you to define a variable that can hold one of several types. This feature is handy for handling situations where a value can take on multiple forms, providing flexibility while maintaining type safety. In this section, we’ll explore how to define and use union types and examples to illustrate their usage.
Defining Union Types
A union type is created using the
Loading...
(pipe) operator to combine multiple types.Loading...
In this example:
- The
Loading...
variable is a union type that can beLoading...
orLoading...
. Loading...
can hold a string or number value, and TypeScript will enforce that it must be one of those types.
Using Union Types with Functions
Union types can be used in function parameters and return types to allow for more flexible function signatures.
Loading...
In this example:
- The
Loading...
function accepts a typeLoading...
parameter. - Inside the function, a type guard (
Loading...
) is used to differentiate between the types and handle each case appropriately.
Union Types with Arrays
Union types can also be used with arrays to allow for collections that can contain multiple types.
Loading...
In this example:
- The
Loading...
array is defined to contain elements that can be eitherLoading...
orLoading...
. - The array can hold a mix of strings and numbers, providing flexibility in the types of elements it can contain.
Complex Union Types
Union types can be combined with other types to create more complex definitions.
Loading...
In this example:
- The
Loading...
interface defines a type with aLoading...
method. - The
Loading...
interface defines a type with aLoading...
method. - The
Loading...
type is a union ofLoading...
andLoading...
, meaning it can be either aLoading...
or aLoading...
. Loading...
is aLoading...
andLoading...
is aLoading...
, each conforming to theLoading...
type.
Narrowing Union Types
When working with union types, type narrowing is essential to ensure correct type handling. TypeScript provides several ways to narrow down union types, including type guards and assertions.
Using Type Guards:
Loading...
In this example:
- The
Loading...
function accepts a typeLoading...
parameter. - The
Loading...
operator is used as a type guard to check if theLoading...
method exists on theLoading...
object, narrowing the type toLoading...
orLoading...
and handling each case appropriately.
Using Type Assertions:
Loading...
In this example:
- Type assertions explicitly assert the type of
Loading...
asLoading...
orLoading...
. - This approach ensures that the correct method is called based on the asserted type.
Union Types with Discriminated Unions
Discriminated unions, also known as tagged unions or algebraic data types, are a powerful pattern for combining union types with a common discriminant property. This approach allows for more precise type narrowing.
Loading...
In this example:
- Both
Loading...
andLoading...
interfaces include aLoading...
property, which acts as the discriminant. - The
Loading...
function uses aLoading...
statement to narrow the type based on theLoading...
property. - This approach provides a clear and type-safe way to handle different cases in a union type.
Union types in TypeScript offer a flexible way to define variables, parameters, and return types that can hold multiple types. By understanding how to define and work with union types, you can create more adaptable and robust TypeScript code. Whether using simple union types, combining them with arrays, or employing discriminated unions for precise type narrowing, union types help you manage complex data structures effectively while maintaining type safety.
Recursive Types in TypeScript
Recursive types in TypeScript allow you to define types that reference themselves, making them ideal for modeling hierarchical or nested data structures, such as trees and linked lists. This section will explore how to define and use recursive types, along with examples to illustrate their usage.
Defining Recursive Types
A recursive type is a type that references itself within its definition. This is commonly done using interfaces or type aliases.
Loading...
In this example:
- The
Loading...
interface defines a type with aLoading...
property and an optionalLoading...
property, an array ofLoading...
objects. - The
Loading...
object is a tree-like structure with nested categories, demonstrating the use of a recursive type.
Using Recursive Types with Type Aliases
Recursive types can also be defined using type aliases.
Loading...
Here, the
Loading...
type alias achieves the same result as the interface example, allowing for recursive structures.Recursive Types for Trees
One everyday use case for recursive types is representing tree structures, such as binary trees or general trees.
Loading...
In this example:
- The
Loading...
interface defines a binary tree node with aLoading...
property and optionalLoading...
andLoading...
properties, which are themselvesLoading...
objects. - The
Loading...
object is a binary tree with nested nodes, demonstrating the use of a recursive type to model a hierarchical structure.
Recursive Types for Linked Lists
Another common use case for recursive types is representing linked lists.
Loading...
In this example:
- The
Loading...
interface defines a linked list node with aLoading...
property and an optionalLoading...
property, which is aLoading...
object. - The
Loading...
object is a linked list with nodes connected through theLoading...
property, demonstrating using a recursive type to model a sequential structure.
Handling Recursive Types with Utility Types
TypeScript provides utility types that can be useful when working with recursive types. For example, you can use the
Loading...
utility type to make all properties of a recursive type optional.Loading...
In this example:
- The
Loading...
type makes all properties of theLoading...
type optional. - The
Loading...
object can have any subset of properties defined in theLoading...
type.
Recursive Type Inference
TypeScript can infer recursive types when defining recursive functions or methods.
Loading...
In this example:
- The
Loading...
function recursively traverses a binary tree and returns an array of node values. - TypeScript correctly infers the recursive type for the
Loading...
parameter and the function's return type.
Recursive types in TypeScript provide a powerful way to model complex hierarchical and nested data structures, such as trees and linked lists. By understanding how to define and use recursive types with
Loading...
andLoading...
aliases, you can create more flexible and robust TypeScript applications. Whether representing tree structures, linked lists, or other recursive data models, recursive types help you manage and traverse these structures effectively while maintaining type safety.TypeScript's type system is a powerful tool for defining and enforcing object structures in your code. You can create robust and maintainable applications by leveraging basic object types, nested types, optional properties, readonly properties, index signatures, intersection types, union types, and recursive types. Understanding these concepts will help you write cleaner, safer, and more expressive TypeScript code. Feel free to experiment with these types in your projects to understand their utility and flexibility better.
Happy coding!
** Book Recommendation:
- React and React Native: A complete hands-on guide to modern web and mobile development with React.js, 3rd Edition
- React Key Concepts
- Pragmatic Programmer The: Your journey to mastery, 20th Anniversary Edition
Mentorship & Consulting - Contact us for more info
Join Our Discord Community Unleash your potential, join a vibrant community of like-minded learners, and let's shape the future of programming together. Click here to join us on Discord.
For Consulting and Mentorship, feel free to contact slavo.io